Custom ActionButtons¶
Keep action buttons visible when scrolling down
Please note that, for technical reasons, the AppGini built-in option "Keep action buttons visible when scrolling down" will not be available when using additional links or buttons.
Bug
Please note there is a bug with AppGini v5.90 and v5.91. See Bugreport and workarounds here.
1 2 |
|
Grouping buttons¶
Additional Action Buttons will be placed at the right hand side below the default Action Buttons (Save, ..., Cancel, Copy).
For technical reasons all additional action buttons have to be grouped.
Groups with title¶
Groups may have a title. You can pass the title to the addGroup()
function as first argument.
1 2 3 4 5 6 7 |
|
Groups without title¶
1 2 3 4 5 6 |
|
Groups with extra css-styling¶
If you want to give the group-header some extra styling, create a custom css class and pass the name of the css class as third parameter:
1 2 3 4 |
|
1 2 3 4 5 6 7 8 9 |
|
Buttons¶
Links to other pages¶
1 2 3 4 5 |
|
Buttons executing javascript functions¶
1 2 3 4 5 6 7 |
|
Variations¶
1 2 3 4 5 6 7 8 9 |
|
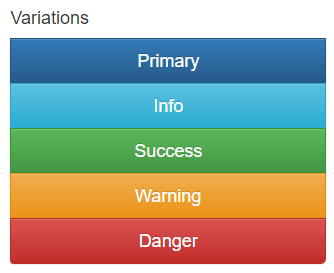
Additional features
Hide button text¶
You can hide the Action Buttons’ text using the .hideText()
method. In combination with the .width()
and .sizeButtons()
methods (see below) this will help you getting more space for data.
js
AppGiniHelper.DV.getActionButtons().hideText();
Button size¶
The .sizeButtons()
method accepts the following values:
Size.lg
Size.md
Size.sm
Size.xs
js
// file: patients-dv.js
var dv = AppGiniHelper.DV;
var actionbuttons = dv.getActionButtons();
actionbuttons.sizeButtons(Size.lg);
Container width¶
You can change the width of the action button container using the following code: ```js // file: patients-dv.js var dv = AppGiniHelper.DV; var actionbuttons = dv.getActionButtons(); actionbuttons.width(2);
// others: // actionbuttons.width(1); // actionbuttons.width(3); ```