Grouping
Screen Recording
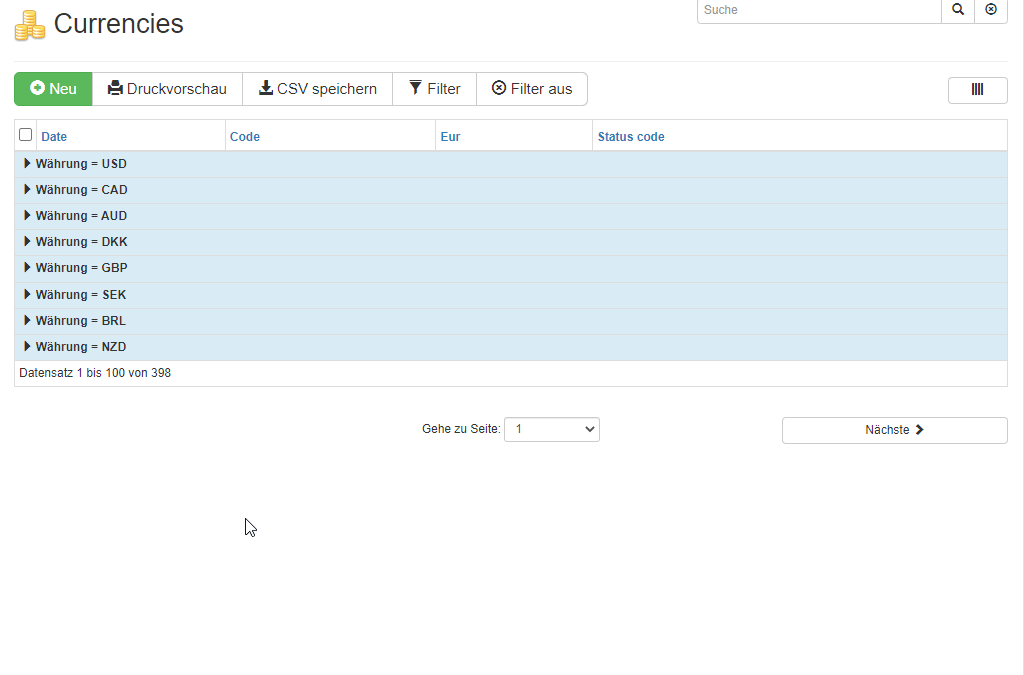
Limitations
Important
This Javascript Library can only work with loaded data in the current page. This function will not fetch all data from the server/database. Grouping will be applied to visible rows, only
Note
Grouping will collide with custom quickfilters (see below). Be careful when combining both, as the results may be mixed up and not clear to the user
Single Column Grouping
TV grouped by currency-code field (named code
) with custom header-caption and collapse/expand
| jQuery(document).ready(function() {
var tv = AppGiniHelper.tv;
let group_by_currency = tv.groupBy("code").setText("Währung");
// apply grouping after adding group-definition(s)
tv.applyGrouping(true);
}
|
Multi-Column Grouping (Nested)
Each group can be (sub-) grouped.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17 | jQuery(document).ready(function() {
var tv = AppGiniHelper.tv;
// level-1: call tv.groupBy()
let group_by_country = tv.groupBy("country_id");
// level-2: based on level-1. call .groupBy() on level-1-variable
let group_by_state = group_by_country.groupBy("state_id");
// level-3 = level-2.groupBy(...)
let group_by_postcode = group_by_state.groupBy("zipcode");
// level-4 = level-3.groupBy(...)
let group_by_city = group_by_postcode.groupBy("city_id");
// apply grouping at the end
tv.applyGrouping(true);
});
|
Extended Grouping
We can customize group-values using functions.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41 | // This is a custom javascript function
// which converts a given text into a date object, if valid,
// using moment-js-library (part of AppGini generated code)
// This function will help us converting strings like "31.12.2021" from the table's cells
// into year ("YYYY") and year-month ("YYYY-MM") strings later on.
function textToDate(text) {
// try to read the input string as a date, formatted as D.M.Y.
// If valid, it returns a new moment-object
return new moment(text, "D.M.Y");
}
jQuery(document).ready(function() {
var tv = AppGiniHelper.tv;
// nested grouping by currency / year / month
// columns: code (currency code like "EUR" or "USD")
// date (string-presentation of date in German formatting like "01.12.2021")
// We are going to use the same column "date" for gruping by year (YYYY) AND for grouping by month (YYYY-MM)
// level 1: group by currency ("code"-column)
// note that we change the header text to "Währung" (which in German means "currency")
let group_by_currency = tv.groupBy("code").setText("Währung");
// level 2: group every currency by year
// note that we pass in a function which converts a given date (string) into a "year"-string
// Additionally we change the header-text to "Jahr" ("Year")
let group_by_year = group_by_currency.groupBy("date", (text) => {
return textToDate(text).format("YYYY");
}).setText("Jahr");
// level 3: group every year by month
// note that we pass in a function which converts the given date (string) into a "year-month"-string
// Additionally we change the header-text to "Monat" ("Month")
let group_by_month = group_by_year.groupBy("date", (text) => {
return textToDate(text).format("YYYY-MM");
}).setText("Monat");
tv.applyGrouping(true);
});
|
Styling
Add a custom CSS style for class .appginihelper-tv-grouping-header
and for .appginihelper-tv-grouping-header .badge
at any place
| .appginihelper-tv-grouping-header {
background-color: black !important;
color: white;
}
.appginihelper-tv-grouping-header .badge {
background-color: white !important;
color: gray;
}
|
Example
1
2
3
4
5
6
7
8
9
10
11
12
13 | <!-- file: hooks/header-extras.php -->
<style>
.appginihelper-tv-grouping-header {
background-color: black !important;
color: white !important;
}
</style>
<?php
// ...
?>
|
There are toolbar buttons for expanding, collapsing and disabling/enabling grouping:
TODO JSE: video with modern style?
Known Issues
- Printing
If you need a Table View printout, the grouped result my not look like what you want
- FIXED 2022/12/20
Due to dynamic generation of additional columns (for groups and subgroups) the column-selection will contain more columns than expected
See also