Multi-Column-Layout¶
Creation¶
Recommended constructor:
1 2 3 |
|
With title:
1 2 |
|
With title and different variation:
1 2 |
|
Add fields to Layout-columns¶
1 2 3 |
|
Alignment of labels and controls¶
Due to Bootstraps 12-column grid-system there may be problems with alignment of labels and controls when using different layouts on the same page.
Parameters¶
- column index, starting at
1
- array (!) of fielnames
Note
Parameter 2 must be an array, not a string.
Deprecated constructor (do not use):
1 2 3 |
|
Please use dv.createLayout()
instead.
Add Multi-Column-Layout to Custom tab¶
1 2 3 4 5 6 7 |
|
Note
When adding layout to a Custom Tab, title will not be rendered;
Mix Layout with fields in Custom Tab¶
1 2 3 4 5 6 7 |
|
Note
When adding layout to a Custom Tab, title will not be rendered;
Example¶
1 2 3 4 |
|
Creates a new row with two columns. Column #1 will have a width of 8
(which means 8/12 ~= 67%), and column #2 will have a width of 4 (which means 4/12 ~= 33%).
Fields last_name
and first_name
will be inserted into column #1
Fields image
and gender
will be inserted into column #2
Alignment: background information¶
Bootstrap’s grid-system provides automatic width calculation for up to 12 columns on different devices. AppGini assigns width of 3/12 of the total column width to labels and 9/12 to controls.
As long as you only have rows of the same width that aligns perfectly as you can see below:
UI design flaw¶
Now the library provides layout functions which allows you to split rows into columns of different widths. You can even mix different layouts per page. As as result there may be flaws with alignment as you can see in this example where we have create first row with width of [12], then second row with widths of [8, 4]:
1 2 3 4 5 6 7 8 9 |
|
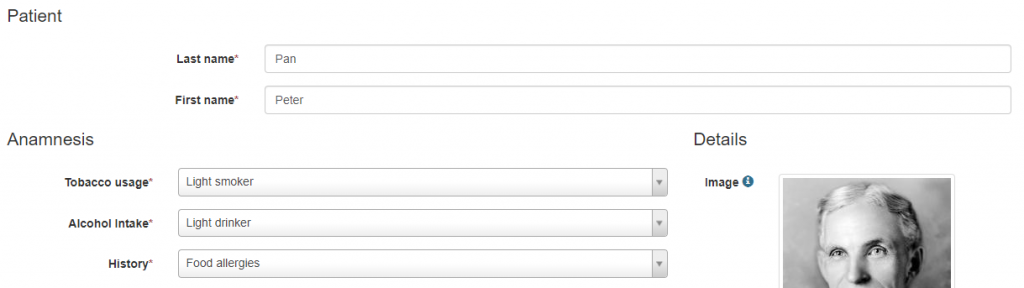
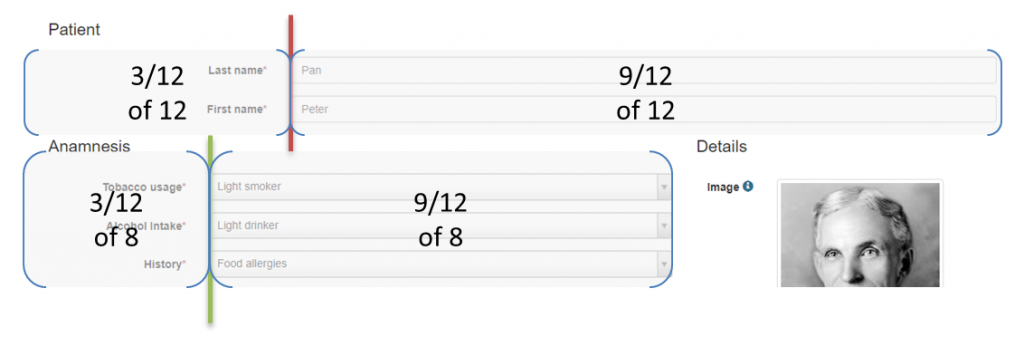
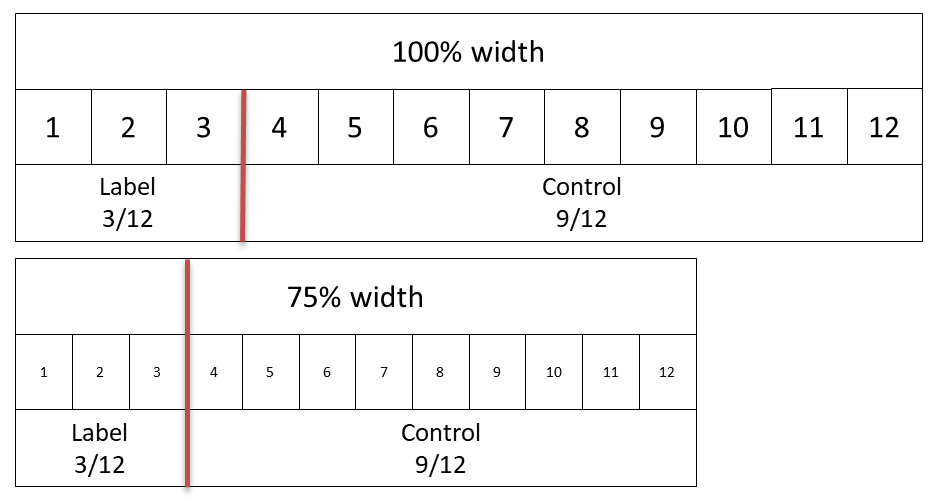
Solutions¶
Our library provides two solutions for these scenarios
Wrapping
You can break label and input into two lines using the wrapLabels()
-function:
1 2 3 4 5 6 7 8 |
|
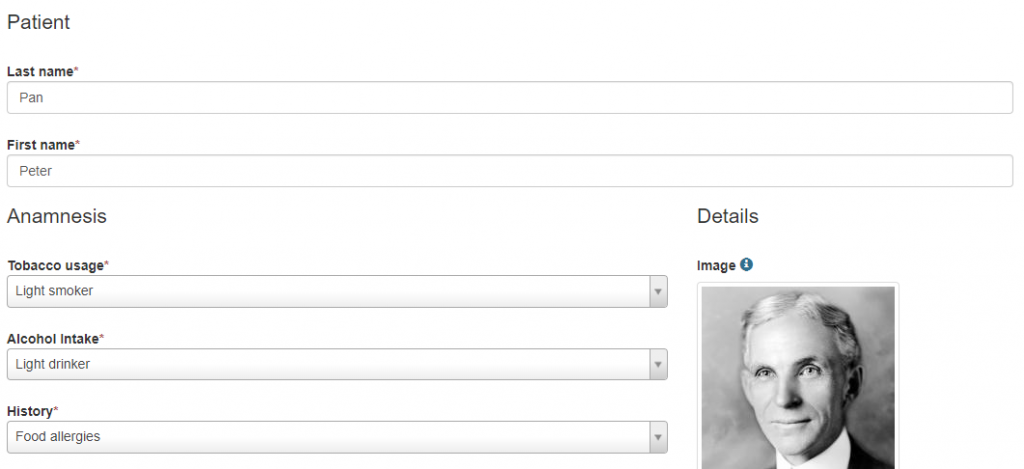
Warning
If you don't have any lookup field (dropdown), the wrapLabel()
function may not work in Add New mode of detail views. If so, please add the following line to your TABLENAME-dv.js file:
1 |
|
Sizing¶
And there is a second option which may result in better results in certain combinations – but not in all.
There is a sizeLabels()
-function you can apply to all labels of a row.
1 2 3 4 5 6 7 8 |
|
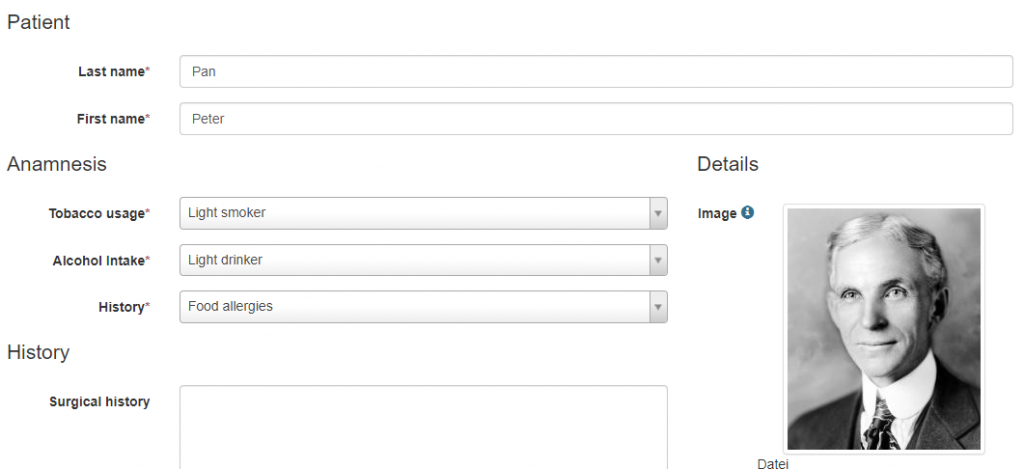
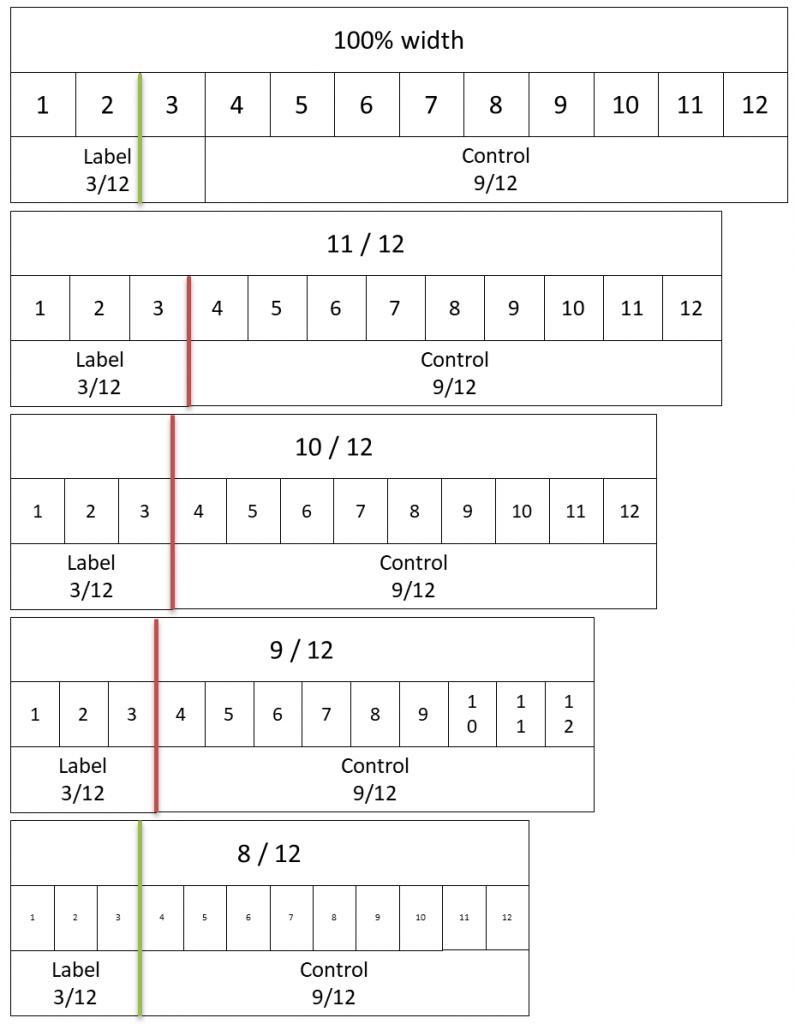
Attention
The sizeLabels()
method will only work for column-widths of 4
, 8
and 12
because in the 12-column grid only 3/12
of 4
, 8
and 12
evaluates to integer values.