Styling Cells
Styling a single column
Fixed style
| jQuery(document).ready(function() {
var tv = AppGiniHelper.tv;
tv.getColumn("COLUMNNAME").style = {
'background-color': 'darkred',
'color': 'white'
};
tv.getColumn("COLUMNNAME").applyStyling();
});
|
Conditional styling
| jQuery(document).ready(function() {
var tv = AppGiniHelper.tv;
tv.getColumn("COLUMNNAME").style = function(text) {
if (text == 'NEW')
return {
'border-left': '4px solid blue;'
};
};
tv.getColumn("COLUMNNAME").applyStyling();
});
|
Conditional styling with comparison-helper
Note the (optional) second argument data
in callback function:
| jQuery(document).ready(function() {
var tv = AppGiniHelper.tv;
tv.getColumn("COLUMNNAME").style = function(text, data) {
if (data.isEmpty()) return {
'background-color': 'red'
};
};
tv.getColumn("COLUMNNAME").applyStyling();
});
|
The data
-argument provides various comparison functions:
(Not) Empty
data.isEmpty()
data.isNotEmpty()
Case insensitive comparison (ignore letter case)
data.equals("search")
data.contains('search')
data.startsWith('prefix')
data.endsWith('suffix')
Case sensitive comparison
data.equals("search", false)
data.contains('search', false)
data.startsWith('prefix', false)
data.endsWith('suffix', false)
Examples
| jQuery(document).ready(function() {
var tv = AppGiniHelper.tv;
tv.getColumn("COLUMNNAME").style = function(text, data) {
if (data.isEmpty()) return {
'background-color': 'red'
};
};
tv.getColumn("COLUMNNAME").applyStyling();
});
|
| jQuery(document).ready(function() {
var tv = AppGiniHelper.tv;
tv.getColumn("COLUMNNAME").style = function(text, data) {
(data.isNotEmpty()) return {
'background-color': 'green'
};
};
tv.getColumn("COLUMNNAME").applyStyling();
});
|
| jQuery(document).ready(function() {
var tv = AppGiniHelper.tv;
tv.getColumn("COLUMNNAME").style = function(text, data) {
if (data.startsWith("A")) return {
'background-color': 'green'
};
};
tv.getColumn("COLUMNNAME").applyStyling();
});
|
| jQuery(document).ready(function() {
var tv = AppGiniHelper.tv;
tv.getColumn("COLUMNNAME").style = function(text, data) {
if (data.endsWith("Z")) return {
'background-color': 'red'
};
};
tv.getColumn("COLUMNNAME").applyStyling();
});
|
| jQuery(document).ready(function() {
var tv = AppGiniHelper.tv;
tv.getColumn("COLUMNNAME").style = function(text, data) {
(data.contains("x")) return {
'background-color': 'blue'
};
};
tv.getColumn("COLUMNNAME").applyStyling();
});
|
Example Output
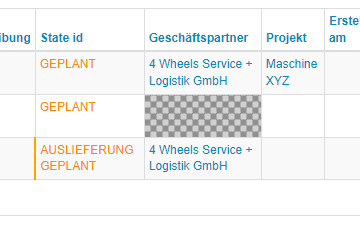
See also